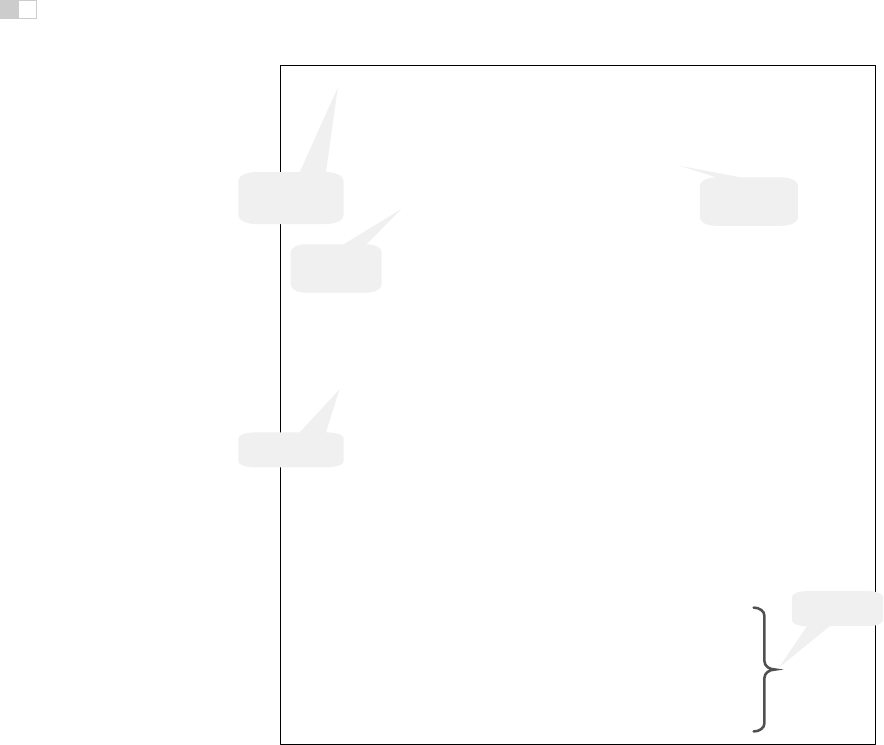
i
i
i
i
i
i
i
i
494 19. Building Interactive Graphics Applications
class ViewController {
private:
ApplicationModel TheModel = null // Reference to the application state
ApplicationView TheView = null // for drawing to the desirable region
public:
void InitializeController(ApplicationMode aModel, anArea) {
// Define and initialize the Application State
TheModel = aModel
TheView = new ApplicationView( anArea )
// Register Event Service Routines
GUISystem::RegisterServiceRoutine(GUISystem:: LMBDown, LMBDownRoutine)
GUISystem::RegisterServiceRoutine(GUISystem:: LMBDrag, LMBDragRoutine)
GUISystem::RegisterServiceRoutine(GUISystem:: LMBUp, LMBUpRoutine)
GUISystem::RegisterServiceRoutine(GUISystem:: RMBDown, RMBDownRoutine)
GUISystem::RegisterServiceRoutine(GUISystem:: RedrawEvent, RedrawRoutine)
}
// Event Service Routines
// … define the 5 event routines similar to the ones in Figure 8 …
}
class GenericController {
private:
ApplicationModel TheModel = null // Reference to the application state
public:
void InitializeController(ApplicationModel aModel, anArea) {
TheModel = aModel
// Register Event Service Routines
GUISystem::RegisterServiceRoutine(GUISystem:: SliderBar, SliderBarRoutine)
GUISystem::DefineTimerPeriod(SimulationUpdateInterval)
GUISystem::RegisterServiceRoutine(GUISystem:: TimerEvent, ServiceTimer)
}
// Event Service Routines
// … define the 2 event routines similar to the ones in Figure 8 …
}
// _______________________________________
// GUI API: MainEventLoop will call this function to initialize our applicaiton
SystemInitialization() {
ApplicationModel
aModel = new ApplicationModel();
ViewController aViewController = new ViewController()
GenericController aGenericController = new GenericController()
aViewController.InitializeController(aModel, drawingAreaOf
Window)
aGenericController.InitializeController(aModel, uiAreaOfWindow)
Creates a new
View for the
s
ecified area
Application
initialization
An area on the
application
windo
Controller with a
View and
Application State
Controller with
no View
Figure 19.13. The controller component of the ball shooting program.
The bottom of Figure 19.13 illustrates that the GUI API MainEventLoop will
still call the SystemInitialization() function to initialize the application. In this
case, we create one instance each of ViewController and GenericController. The
ViewController is initialized to monitor mouse button events in the drawing area
of the application window (e.g., LMB click to define HeroBall), while the Gener-
icController is initialized to monitor the GUI element state changes (e.g., LMB
dragging of a slider bar). Notice that the service of the timer event is global to the
entire application and should be defined in only one of the controllers (either one
will do).
In practice, the GUI API MainEventLoop dispatches events to the controllers
based on the context of the event. The context of an event is typically defined by