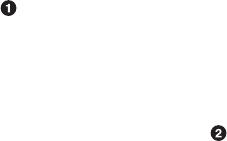
Before you get started, make sure that both the gpiozero and
OpenCV Python libraries are installed and working properly on
your Raspberry Pi.
1. As you did in Chapter 7, connect pin 24 to the pushbutton.
One side of the button should be connected to ground, the other
to pin 24. (Remember, we’re talking about BCM numbers here,
not physical pins. You’re actually connecting to physical pin #18
on the Pi.)
2. Find or create a small image of a black mustache on a white
background and save it as
moustache.png
in a new folder called
photobooth
on your Raspberry Pi. You can also download a pre-
made mustache file from the
images
subdirectory of the Github
repository for this book: github.com/wdonat/gsw_raspi_4e.
3. From the same repository, grab the haarcascade_mcs_mouth.
xml file and place it into the
photobooth
directory. (If you
download the file from Github’s web interface, the best way
to do it is to get the raw version of the file, copy all of the text,
and then paste it into a blank text document on your computer.
Then save as haarcascade_mcs_mouth.xml.)
4. In the
photobooth
directory, create a new file called
photo-booth.py
, type in the code listed in Example 9-6, and save
the file.
Example 9-6. Source code for photobooth.py
import cv2
from picamera.array import PiRGB Array
from picamera import PiCamera
from gpiozero import Button
import time
button = Button(24)
camera = PiCamera()
camera.resolution = (800, 608)
rawCapture = PiRGBArray (camera)
mouth_cascade =
cv2.CascadeClassier(‘'haarcascade_mcs_mouth.xml')
162 Getting Started with Raspberry Pi
GSW_RASPI_4ED_FIN.indd 162GSW_RASPI_4ED_FIN.indd 162 10/28/21 10:54 AM10/28/21 10:54 AM