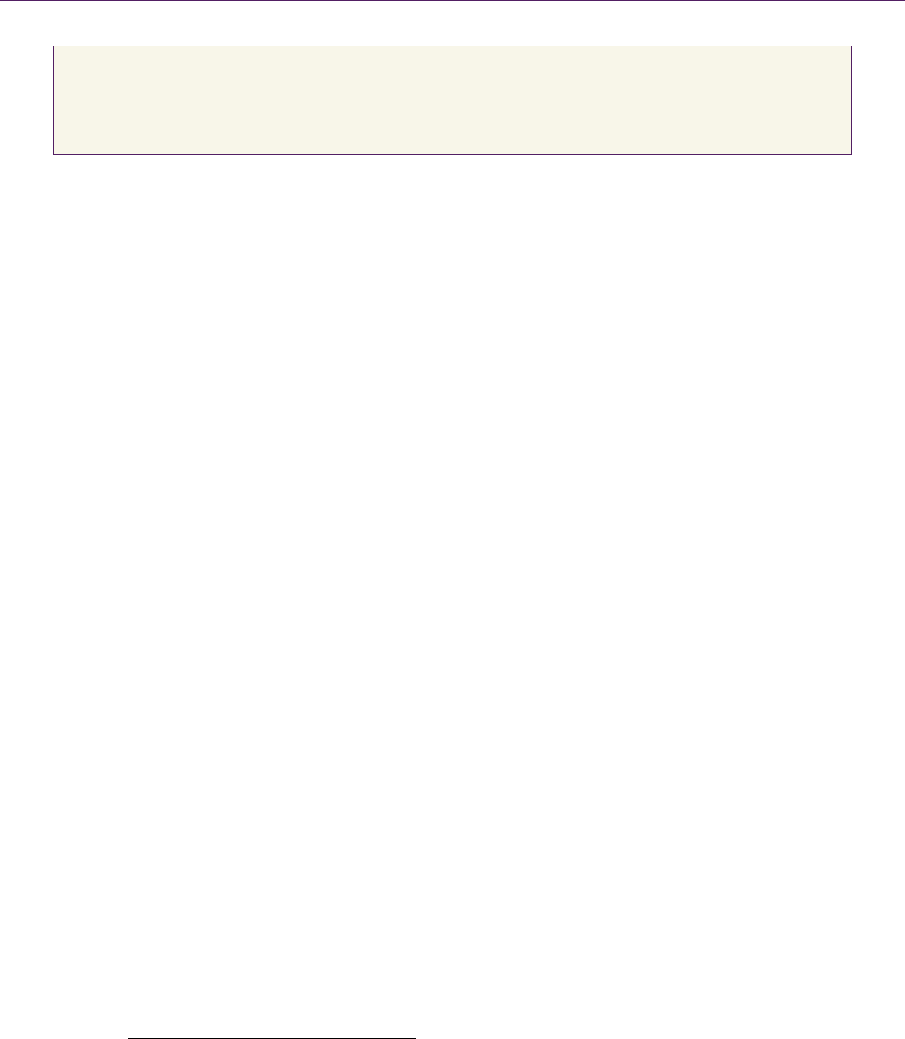
6.4BeyondGLSLBuilt‐inUniforms 95
std::for_each(m_dirtyUniforms.begin(), m_dirtyUniforms.end(),
std::mem_fun(&ICleanable::Clean));
m_dirtyUniforms.clear();
}
Listing 6.8. A modified ShaderProgram::Clean() method that automatically sets engine
uniforms.
6.4BeyondGLSLBuilt‐inUniforms
Thus far, we’ve focused on using our engine uniform framework to replace the
functionality of the old GLSL built-in uniforms. Our framework extends far be-
yond this, however. Its true usefulness is shown when engine uniforms based on
higher-level engine or application-specific data are added. For example, a planet-
based game may have engine uniforms for the sun position or the player’s alti-
tude. Since engine uniforms are defined using interfaces, new engine uniforms
can be added with very little impact.
These high-level engine uniforms can include things like the current time or
frame number, which are useful in animation. For example, texture animation
may be implemented by translating texture coordinates in a fragment shader
based on a uniform containing the current time. To use the uniform, the shader
author doesn’t have to do any additional work other than define the uniform.
If the
ShaderProgram::InitializeEngineUniforms() method is coded
carefully, applications that have access to headers for
IEngineUniform and
IEngineUniformFactory, but not necessarily access to all of the engine’s source
code, can also add engine attributes.
Engine uniforms can even go beyond setting uniform values. In Insight3D,
1
we use engine uniforms to provide shaders access to the scene’s depth and sil-
houette textures. In these cases, the implementation of the
IEngineUni-
form::Set()
method binds a texture to a texture unit instead of actually setting a
uniform’s value (the value for the
sampler2D uniform is set just once to the tex-
ture unit index in the engine uniform’s constructor). It is also common for en-
gines to provide engine uniforms for noise textures.
6.5ImplementationTips
To reduce the amount of code required for new engine uniforms, it is possible to
use C++ templates to let the compiler write a factory for each engine uniform for
1
See http://www.insight3d.com/.