Create the following file structure inside the courses application directory:
templates/
base.html
registration/
login.html
logged_out.html
Before building the authentication templates, we need to prepare the base template for our project. Edit the base.html template file and add the following content to it:
{% load staticfiles %}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>{% block title %}Educa{% endblock %}</title>
<link href="{% static "css/base.css" %}" rel="stylesheet">
</head>
<body>
<div id="header">
<a href="/" class="logo">Educa</a>
<ul class="menu">
{% if request.user.is_authenticated %}
<li><a href="{% url "logout" %}">Sign out</a></li>
{% else %}
<li><a href="{% url "login" %}">Sign in</a></li>
{% endif %}
</ul>
</div>
<div id="content">
{% block content %}
{% endblock %}
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/
3.3.1/jquery.min.js"></script>
<script>
$(document).ready(function() {
{% block domready %}
{% endblock %}
});
</script>
</body>
</html>
This is the base template that will be extended by the rest of the templates. In this template, we define the following blocks:
- title: The block for other templates to add a custom title for each page.
- content: The main block for content. All templates that extend the base template should add content to this block.
- domready: Located inside the $document.ready() function of jQuery. It allows us to execute code when the DOM has finished loading.
The CSS styles used in this template are located in the static/ directory of the courses application, in the code that comes along with this chapter. Copy the static/ directory into the same directory of your project to use them.
Edit the registration/login.html template and add the following code to it:
{% extends "base.html" %}
{% block title %}Log-in{% endblock %}
{% block content %}
<h1>Log-in</h1>
<div class="module">
{% if form.errors %}
<p>Your username and password didn't match. Please try again.</p>
{% else %}
<p>Please, use the following form to log-in:</p>
{% endif %}
<div class="login-form">
<form action="{% url 'login' %}" method="post">
{{ form.as_p }}
{% csrf_token %}
<input type="hidden" name="next" value="{{ next }}" />
<p><input type="submit" value="Log-in"></p>
</form>
</div>
</div>
{% endblock %}
This is a standard login template for Django's login view.
Edit the registration/logged_out.html template and add the following code to it:
{% extends "base.html" %}
{% block title %}Logged out{% endblock %}
{% block content %}
<h1>Logged out</h1>
<div class="module">
<p>You have been successfully logged out.
You can <a href="{% url "login" %}">log-in again</a>.</p>
</div>
{% endblock %}
This is the template that will be displayed to the user after logout. Run the development server with the following command:
python manage.py runserver
Open http://127.0.0.1:8000/accounts/login/ in your browser. You should see the login page like this:
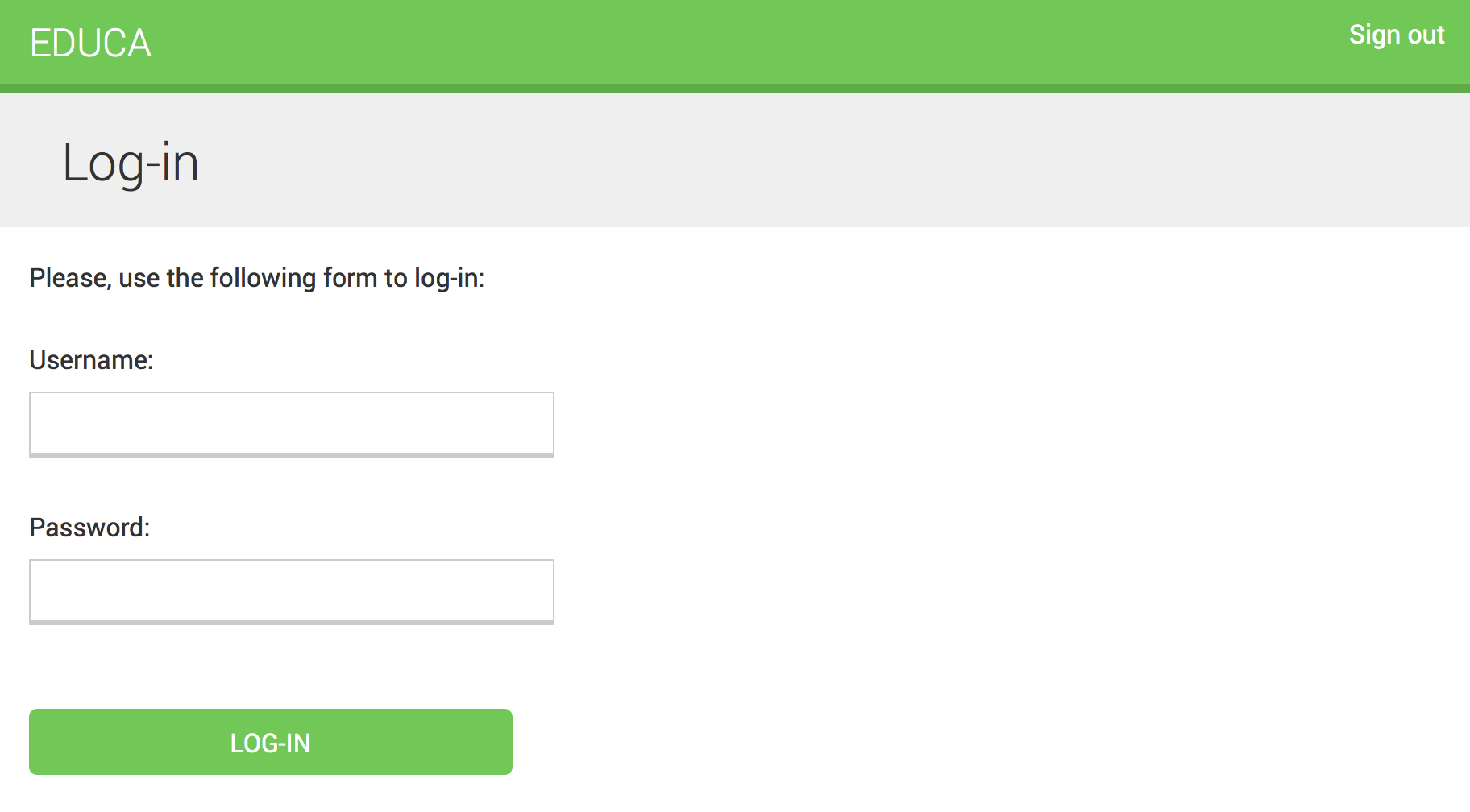